摘要
在現代軟件開發中,準確處理不同單位的轉換是一個常見而復雜的需求。無論是處理溫度、長度、重量還是其他物理量,都需要可靠的單位轉換機制。本文將深入介紹 Units.NET 庫,展示如何在 .NET 應用中優雅地處理單位轉換。
基礎配置
首先,通過 NuGet 安裝 Units.NET:
| <PackageReference Include="UnitsNet" Version="5.x.x" /> |
實戰示例:天氣 API
基礎模型定義
| public record WeatherForecast( |
| Temperature Temperature, |
| DateTime Date, |
| string Summary |
| ); |
|
|
| public record WeatherResponse( |
| string DisplayValue, |
| DateTime Date, |
| string Summary |
| ); |
API 端點實現
| var summaries = new[] |
| { |
| "Freezing", "Bracing", "Chilly", "Cool", "Mild", "Warm", "Balmy", "Hot", "Sweltering", "Scorching" |
| }; |
|
|
| app.MapGet("/weather", (string? unit) => |
| { |
| var forecasts = Enumerable.Range(1, 5).Select(index => |
| { |
| |
| var tempC = Temperature.FromDegreesCelsius(Random.Shared.Next(-20, 55)); |
| |
| var temp = unit?.ToLowerInvariant() switch |
| { |
| "f" or "fahrenheit" => tempC.ToUnit(TemperatureUnit.DegreeFahrenheit), |
| "k" or "kelvin" => tempC.ToUnit(TemperatureUnit.Kelvin), |
| _ => tempC |
| }; |
|
|
| return new WeatherForecast( |
| Temperature: temp, |
| Date: DateTime.Now.AddDays(index), |
| Summary: summaries[Random.Shared.Next(summaries.Length)] |
| ); |
| }) |
| .ToArray(); |
|
|
| return forecasts.Select(f => new WeatherResponse( |
| Date: f.Date, |
| Summary: f.Summary, |
| DisplayValue: f.Temperature.ToString("F2") |
| )); |
| }) |
| .WithName("GetWeatherForecast"); |
當請求的units單位不同時,將輸出相同溫度的不同單位表示:
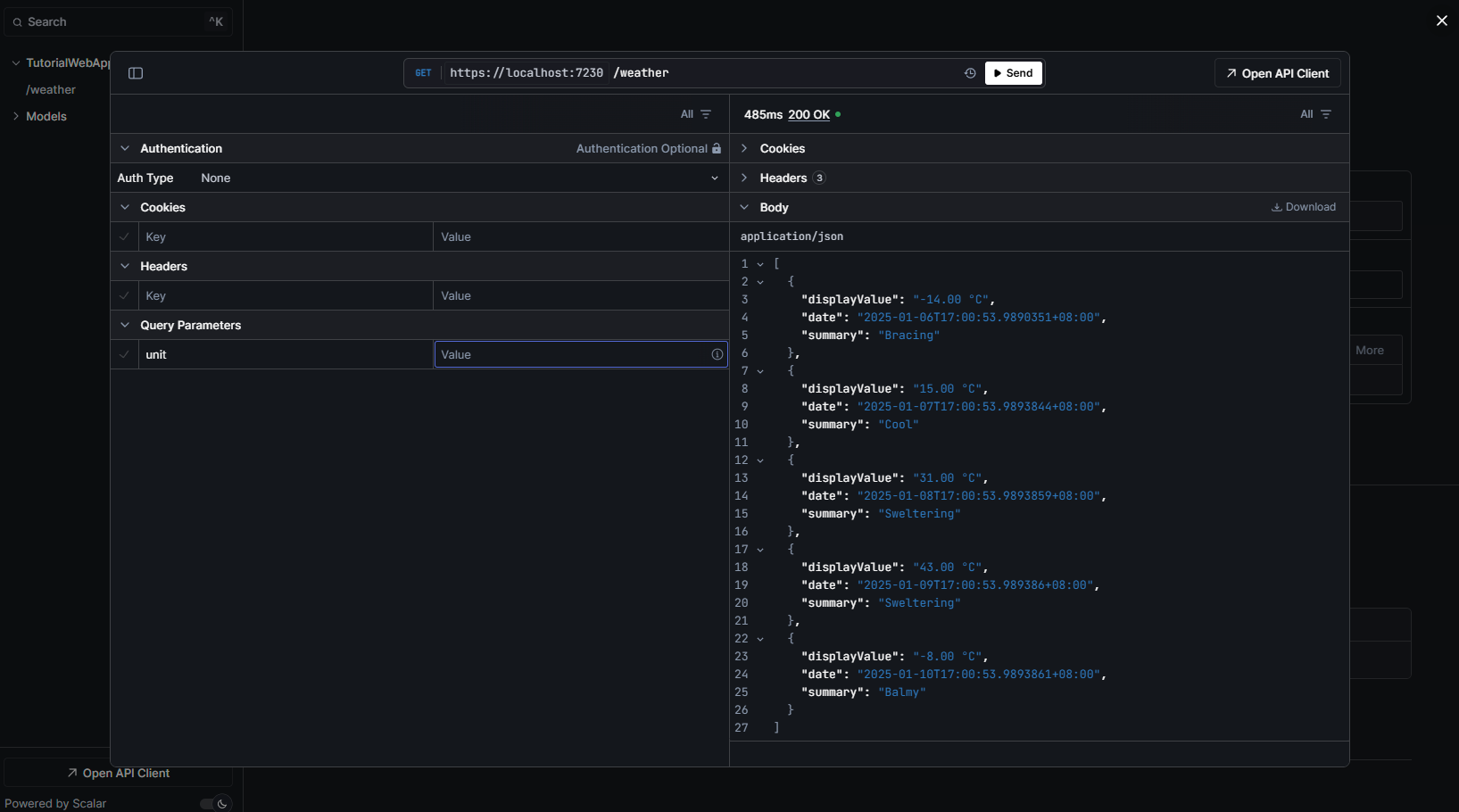
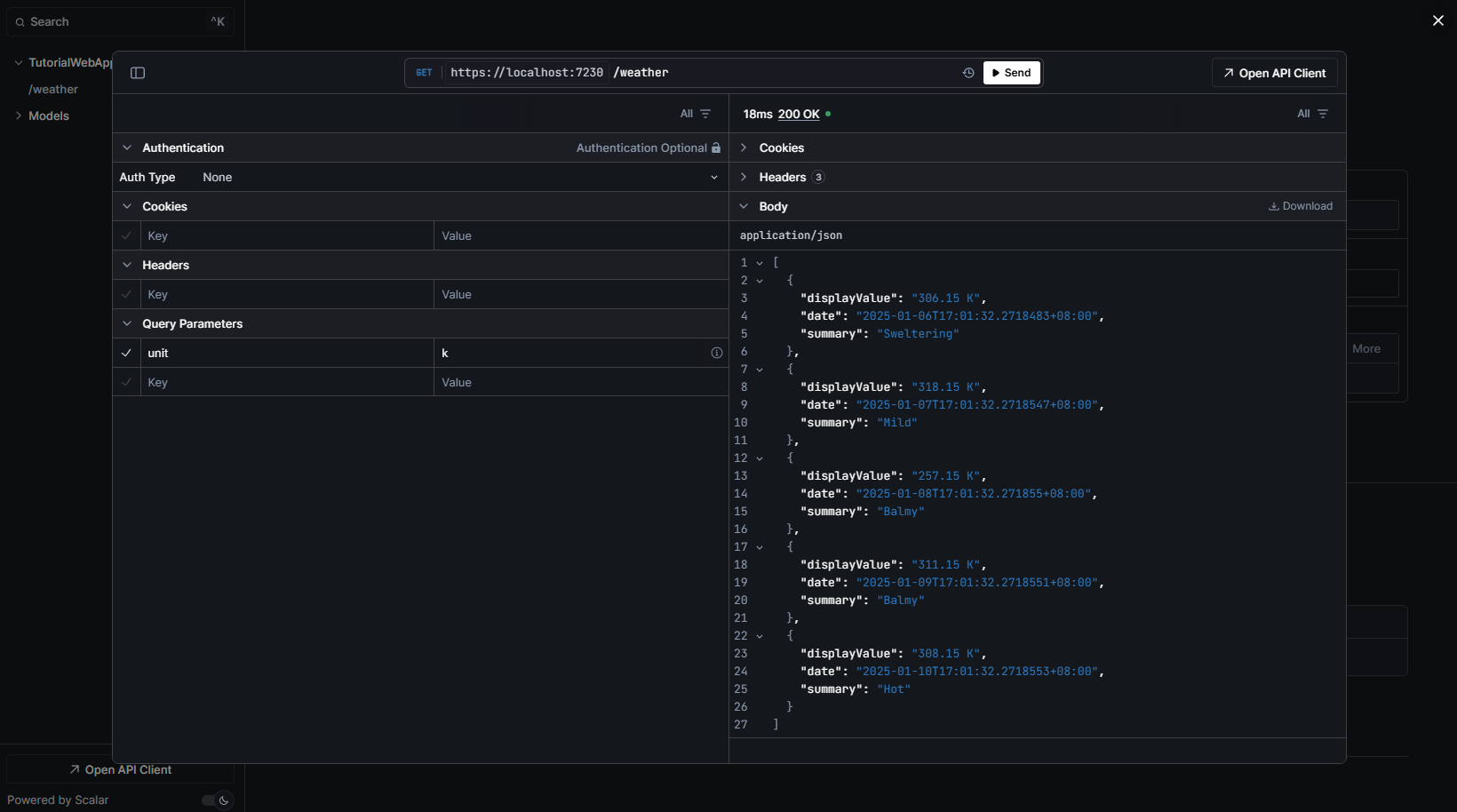
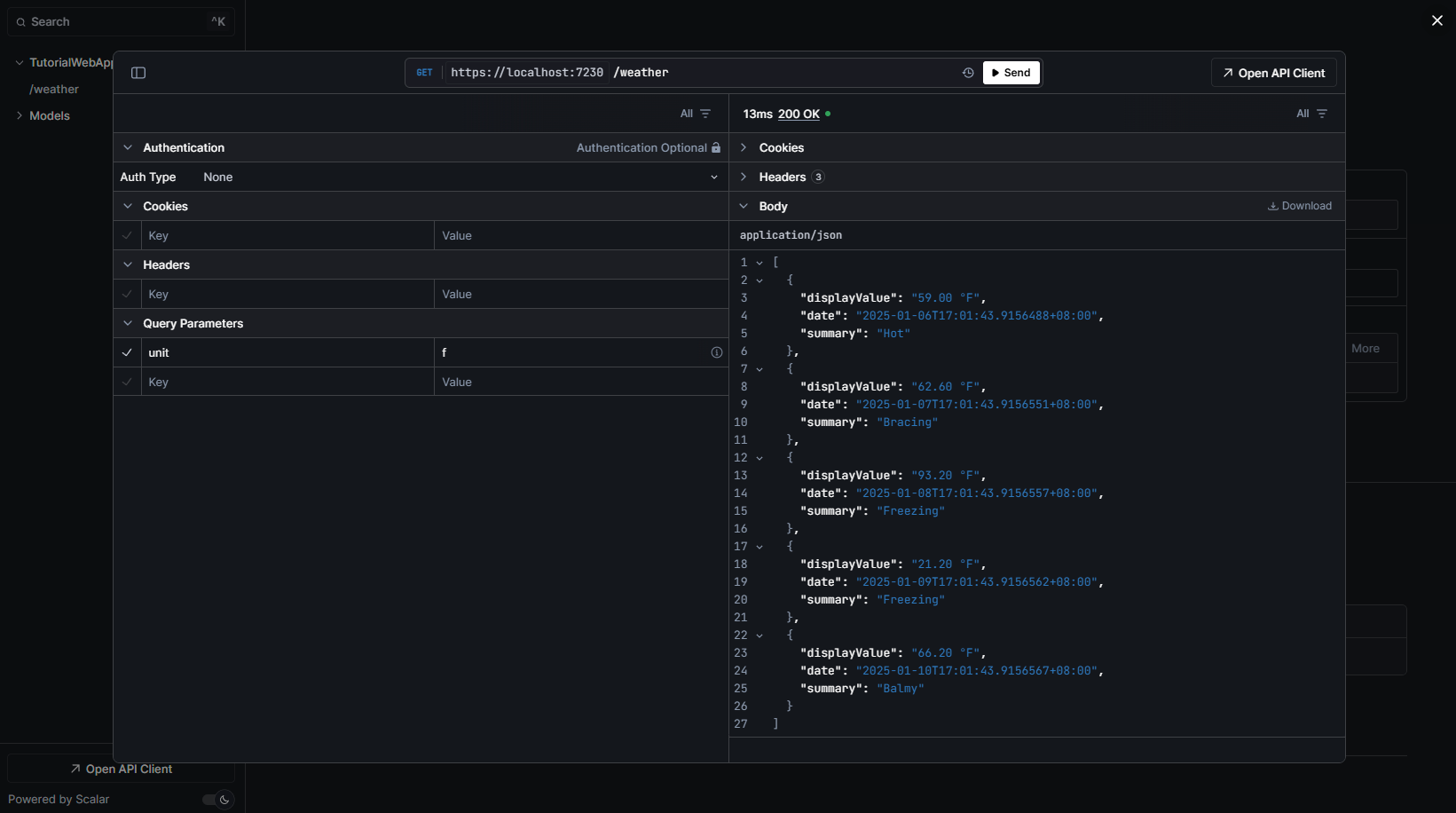
單位相互轉換
| public static class UnitConverter |
| { |
| public static Temperature ConvertTemperature( |
| double value, |
| string fromUnit, |
| string toUnit) |
| { |
| var temperature = fromUnit.ToLowerInvariant() switch |
| { |
| "c" => Temperature.FromDegreesCelsius(value), |
| "f" => Temperature.FromDegreesFahrenheit(value), |
| "k" => Temperature.FromKelvins(value), |
| _ => throw new ArgumentException($"Unsupported unit: {fromUnit}") |
| }; |
|
|
| return toUnit.ToLowerInvariant() switch |
| { |
| "c" => temperature.ToUnit(TemperatureUnit.DegreeCelsius), |
| "f" => temperature.ToUnit(TemperatureUnit.DegreeFahrenheit), |
| "k" => temperature.ToUnit(TemperatureUnit.Kelvin), |
| _ => throw new ArgumentException($"Unsupported unit: {toUnit}") |
| }; |
| } |
| } |
數學運算支持
Units.NET 支持各種數學運算,使得單位計算變得簡單:
| public class UnitCalculations |
| { |
| public static Speed CalculateSpeed(Length distance, Duration time) |
| { |
| return distance / time; |
| } |
|
|
| public static Acceleration CalculateAcceleration(Speed initialSpeed, Speed finalSpeed, Duration time) |
| { |
| return (finalSpeed - initialSpeed) / time; |
| } |
|
|
| public static Energy CalculateKineticEnergy(Mass mass, Speed velocity) |
| { |
| double massValue = mass.Kilograms; |
| double velocityValue = velocity.MetersPerSecond; |
| double energyValue = 0.5 * massValue * velocityValue * velocityValue; |
| return Energy.FromJoules(energyValue); |
| } |
| } |
|
|
| |
| var distance = Length.FromKilometers(100); |
| var time = Duration.FromHours(2); |
| var speed = UnitCalculations.CalculateSpeed(distance, time); |
| Console.WriteLine($"Speed: {speed.ToUnit(SpeedUnit.KilometerPerHour)}"); |
代碼執行后,控制臺將輸出:Speed: 50 km/h
文化本地化支持
| var usEnglish = new CultureInfo("en-US"); |
| var russian = new CultureInfo("ru-RU"); |
| var oneKg = Mass.FromKilograms(1); |
| |
| |
| CultureInfo.CurrentCulture = russian; |
| string kgRu = oneKg.ToString(); |
|
|
| |
| string mgUs = oneKg.ToUnit(MassUnit.Milligram).ToString(usEnglish); |
| string mgRu = oneKg.ToUnit(MassUnit.Milligram).ToString(russian); |
|
|
| Console.WriteLine(mgUs); |
| Console.WriteLine(mgRu); |
| |
| Mass kg = Mass.Parse("1.0 kg", usEnglish); |
|
|
| |
| RotationalSpeedUnit rpm1 = RotationalSpeed.ParseUnit("rpm"); |
| RotationalSpeedUnit rpm2 = RotationalSpeed.ParseUnit("r/min"); |
|
|
| |
| string kgAbbreviation = Mass.GetAbbreviation(MassUnit.Kilogram); |
控制臺將輸出不同文化設置下的標準單位
結論
Units.NET 是一個強大而靈活的單位轉換庫,它不僅簡化了單位轉換的實現,還提供了豐富的功能支持。通過使用 Units.NET,開發者可以專注于業務邏輯,而不必擔心單位轉換的復雜性。無論是構建天氣 API、物流系統還是科學計算應用,Units.NET 都是處理單位轉換的理想選擇。
轉自https://www.cnblogs.com/madtom/p/18653522
該文章在 2025/1/7 15:42:57 編輯過