數組是一種基本的數據結構,用于在單個變量下存儲固定大小的相同類型元素的順序集合。在 C# 中,數組是一種引用類型,可以用于存儲多個數據項。
理解數組
數組的定義
數組是一種數據結構,它可以存儲一系列相同類型的元素。在 C# 中,數組的索引從 0 開始。
數組的聲明
在 C# 中,聲明數組的語法如下:
dataType[] arrayName;
例如:
int[] numbers;
string[] names;
數組的初始化
數組的初始化可以在聲明時進行,也可以在聲明后進行。
int[] numbers = new int[5]; // 聲明一個包含5個整數的數組
string[] names = { "Alice", "Bob", "Charlie" }; // 聲明并初始化一個字符串數組
數組的訪問
訪問數組中的元素通過索引進行:
int firstNumber = numbers[0]; // 訪問第一個元素
string firstNames = names[0]; // 訪問第一個名字
數組的特點
固定大小
一旦數組被創建,它的大小就是固定的,不能增加或減少。
同類型元素
數組只能存儲同一數據類型的元素。
隨機訪問
數組支持通過索引隨機訪問元素,訪問速度快。
數組的應用場景
數據集合
當你需要存儲一組相同類型的數據時,如學生的成績列表。
緩存機制
當你需要緩存數據以便快速訪問時,如圖像處理中的像素數據。
查找表
當你需要通過索引快速查找數據時,如鍵盤字符與其 ASCII 值的映射。
臨時存儲
當你需要臨時存儲數據進行處理時,如排序算法中的輔助數組。
數組的例子
基本數組操作
namespace App08
{
internal class Program
{
static void Main(string[] args)
{
// 聲明并初始化一個整數數組
int[] scores = newint[] { 90, 85, 80, 75, 70 };
// 遍歷數組
for (int i = 0; i < scores.Length; i++)
{
Console.WriteLine(scores[i]);
}
// 使用 foreach 遍歷數組
foreach (int score in scores)
{
Console.WriteLine(score);
}
// 修改數組元素
scores[2] = 82;
// 多維數組
int[,] matrix = newint[3, 3] {
{ 1, 2, 3 },
{ 4, 5, 6 },
{ 7, 8, 9 }
};
// 遍歷二維數組
for (int i = 0; i < matrix.GetLength(0); i++)
{
for (int j = 0; j < matrix.GetLength(1); j++)
{
Console.Write(matrix[i, j] + " ");
}
Console.WriteLine();
}
}
}
}
?
數組作為方法參數
namespace App08
{
internal class Program
{
static void Main(string[] args)
{
// 定義一個計算數組元素總和的方法
static int Sum(int[] arr)
{
int sum = 0;
foreach (int item in arr)
{
sum += item;
}
return sum;
}
// 調用方法
int[] numbers = { 1, 2, 3, 4, 5 };
int total = Sum(numbers);
Console.WriteLine("Total: " + total);
}
}
}
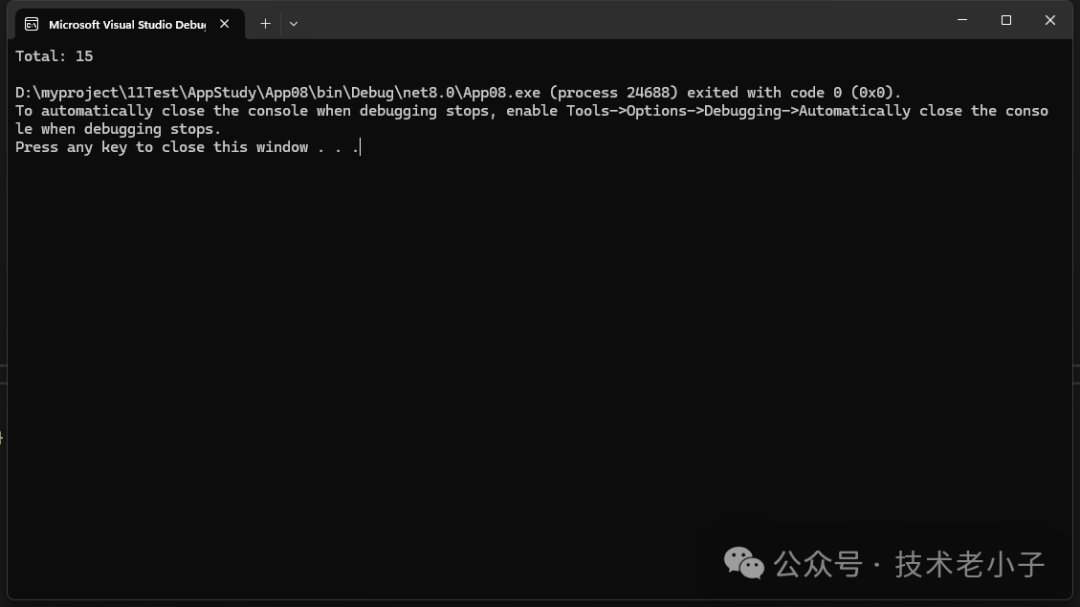
數組的排序和搜索
namespace App08
{
internal class Program
{
static void Main(string[] args)
{
// 排序數組
int[] nums = { 3, 1, 4, 1, 5, 9 };
Array.Sort(nums);
// 搜索數組
int index = Array.IndexOf(nums, 4);
Console.WriteLine("Index of 4: " + index);
}
}
}
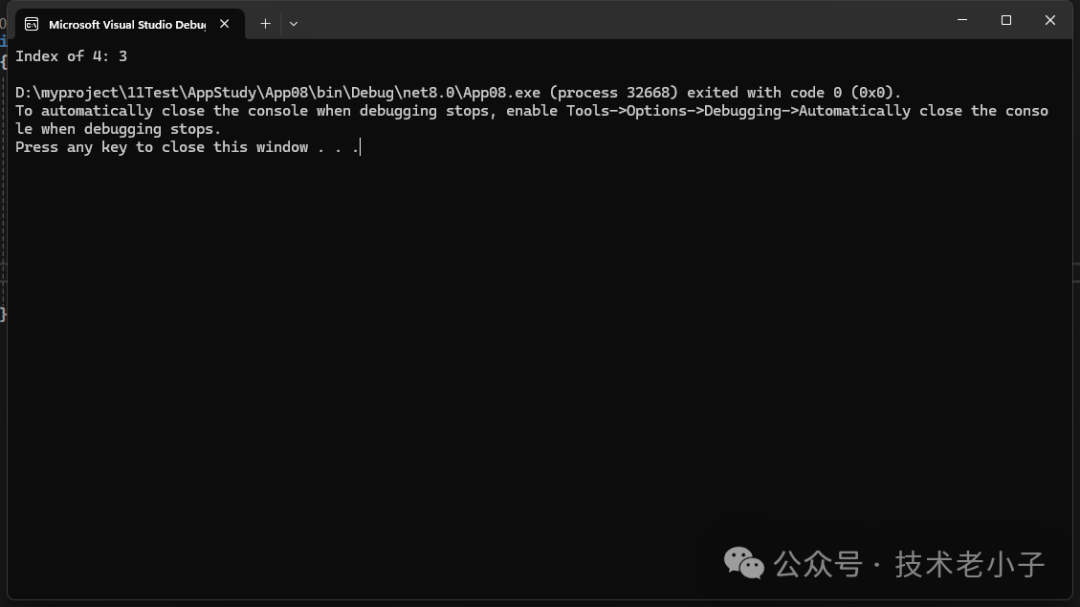
總結
數組是 C# 中一種非常重要的數據結構,它提供了一種有效的方式來存儲和訪問一系列相同類型的數據。雖然數組具有固定大小的限制,但它們在許多編程情境中是不可或缺的,特別是在需要快速按索引訪問數據時。通過本課程的學習,你應該能夠理解數組的基本概念、特點和應用場景,并能夠在 C# 程序中有效地使用數組。
閱讀原文:原文鏈接
該文章在 2025/3/24 17:21:01 編輯過